Video calls can be recorded using the Web user control
Recording requires the user to provide a valid "Recording Session" configuration (check the "Recording Configuration" section below).
Once this property is properly configured, the video call can be recorded simply by calling the user control 's "StartRecording()" method.
This can be invoked at any time:
e.g.1: on-demand, after a button is pressed
Event 'RECButtonPressed'
DVSinchClientWeb1.StartRecording()
EndEvent
e.g.2: Start recording automatically after the video call is established
Event DVSinchClientWeb1.onCallEstablished
DVSinchClientWeb1.StartRecording()
EndEvent
The recording can be manually stopped at any time, by invoking the "StopRecording()" method.
Event 'StopRECPressed'
DVSinchClientWeb1.StopRecording()
EndEvent
However, if the video call stops or fails, the recording is automatically stopped.
Once the recording starts, it will fire the following events:
This event is fired every time a video "part" is generated.
This event is fired if the video call could not be recorded.
This event is fired when the recorded video is uploaded to the server.
This event is fired if the video could not be uploaded to the server.
To enable video call recording, the property "Recording Session" must be set.
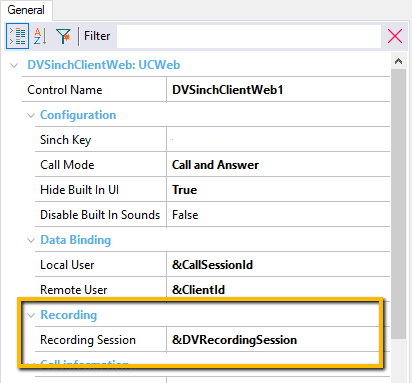
The user must configure the recording settings using the SDT named "DVRecordingSession".
A sample configuration is distributed in the procedure "DVGetRecordingSessionSample"
Event Start
&DVRecordingSession = DVGetRecordingSessionSample(true, false)
EndEvent
But the user can configure the recording anywhere
e.g.:
Event Start
//Recording Settings
&DVRecordingSession.RecordingType = DVRecordingType.Local
&DVRecordingSession.RecordingFileName = !"myRecording"
&DVRecordingSession.RecordingMimeType = DVRecordingMimeType.webm
//Local Recording Settiongs
&DVRecordingSession.LocalRecording.SaveInterval = 0 // Will save 1 video for the Entire Session (at the end of the session)
//Audio and video Quality
&DVRecordingSession.RecordingQuality.AudioBitrate = 256
&DVRecordingSession.RecordingQuality.VideoBitrate = 1024
//Custom Data
&DVRecordingSession.RecordingMetadata.Id = !"1" //Something that identifies the Session
&DVRecordingSession.RecordingMetadata.Data = !"My First Recording from Web Interface using DV Usercontrol"
EndEvent
&DVRecordingSession.RecordingQuality.VideoBitrate = 64
&DVRecordingSession.RecordingQuality.AudioBitrate = 32
&DVRecordingSession.RecordingQuality.VideoAudioQuaility = 0
&DVRecordingSession.RecordingQuality.VideoWidth = 320
&DVRecordingSession.RecordingQuality.VideoHeight = 240
&DVRecordingSession.RecordingQuality.VideoBitrate = 128
&DVRecordingSession.RecordingQuality.AudioBitrate = 64
&DVRecordingSession.RecordingQuality.VideoAudioQuaility = 0
&DVRecordingSession.RecordingQuality.VideoWidth = 640
&DVRecordingSession.RecordingQuality.VideoHeight = 480
The result of recording a video call will be a new video in "webm" or "mkv" format. Please check the formats section for details.
This video will contain both video sources (local and remote) "side by side", where the remote video is placed on the left, and local video will be placed on the right.
The resulting video can be either generated and downloaded locally as a direct Http download, or the video can be automatically uploaded to the server where it can be processed and stored.
This can be configured using the "RecordingType" setting
&DVRecordingSession.RecordingType = DVRecordingType.Local
Prompts the user to save the file inside the web browser, as a common Http download.
In this case, the video will be downloaded in ".webm" format. Please check the formats section for details.
&DVRecordingSession.RecordingType = DVRecordingType.Remote
&DVRecordingSession.RemoteRecording.SaveInterval = 10 //Video part will be sent every 10 seconds
&DVRecordingSession.RemoteRecording.ServerEndpointURL = DVStreamReceiverBinary.Link() //Http Handler
Every "SaveInterval" seconds, the video buffer binary is sent to the Server. Every video part is saved in the webserver and when recording stops, video parts are joined.
Videos are saved inside Folder "PublicTempStorage/videos" of the WebApp. (Configurable in Object Procedure 'DVRecordingUploadPath')
In this case, the video will be downloaded in ".mkv" format. Please check the formats section for details.
Once video recording is completed, the "onRecordingRemoteUploadEnded" is fired, and the URL of the resulting video is provided:
Event DVSinchClientWeb1.onRecordingRemoteUploadEnded
&UploadedVideoUrl = &DVRecordingSession.Session.RemoteResponse.resourceUrl
MSG("Video uploaded to: " + &UploadedVideoUrl)
EndEvent
At this point, the video can be processed or stored in the server using a GeneXus Transaction.
e.g.:
&RecordingSession.RecordingSessionId.FromString(&DVRecordingSession.RecordingMetadata.Id)
&RecordingSession.RecordingSessionFinished = true
&RecordingSession.RecordingSessionVideo.VideoURI = &DVRecordingSession.Session.RemoteResponse.resourceUrl
&RecordingSession.RecordingSessionError = &DVRecordingSession.Session.RemoteResponse.success
&RecordingSession.Update()
commit
An export file containing this sample "RecordingSession" transaction can be downloaded here.
To complete the sample, the user must include the following code:
Event DVSinchClientWeb1.onCallEstablished
DVSinchClientWeb1.StartRecording()
StartRecordingSession(&DVRecordingSession)
EndEvent
Event DVSinchClientWeb1.onRecordingRemoteUploadEnded
EndRecordingSession(&DVRecordingSession)
msg(!"Recording Upload Success")
msg(&DVRecordingSession.Session.RemoteResponse.resourceUrl)
EndEvent
Event DVSinchClientWeb1.onRecordingRemoteUploadFailed
EndRecordingSession(&DVRecordingSession)
msg(!"Recording Failed")
EndEvent
- .NET
- .NET Core
- Java (Windows)
- Java (Linux) *
* Recorded videos are not seekable.
- Every error is written to the User Log, using the built-in API of GeneXus.
- Video parts are joined using: mkvmerge.exe (Windows) or 'FileStreamBinary' (Linux)
When using the local recording, the video will be generated in ".webm" format:
- This format is standard and will work in most commercial video players across operating systems: VLC, GOM, etc.
- ".webm" files can also be opened using any modern Web browser (Chrome, Firefox, Edge Chromium, etc)
- Other Video Players (like the Win32 application "Windows media player") may require additional "codec" installation (please check official documentation https://www.webmproject.org/tools/)
- This video format is not currently compatible with Windows 10 "Movies and TV" UWP application.
When using the remote recording, the video will be generated in ".mkv" format:
- Mkv is an open-source format that will work in most commercial video players across operating systems: VLC, GOM, etc.
- Other Video Players (like the Win32 application "Windows media player") may require additional "codec" installation.
- This video format is compatible with Windows 10 "Movies and TV" UWP application.
IIS default max upload size is limited to 2 MB. This can provoke the error: '413.1 Content-Length Too Large'
To prevent this error, add to 'web.config' file the attribute 'maxRequestLength="1048576"'
<configuration>
...
<system.web>
...
<httpRuntime maxRequestLength="1048576" />
Videocalls are recorded as '.mkv' files, so the 'video/mkv' MIME-Type is required for the Server to Serve the Final video through HTTP.
In the 'web.config' file, add the corresponding 'mimeMap' under the section 'staticContent':
<configuration>
...
<system.webServer>
...
<staticContent>
...
<mimeMap fileExtension=".mkv" mimeType="video/mkv" />
|