WorkWithPlus for VideoCall 5 requires some migrations in the GeneXus code:
Step 1: Install the new version
Use the setup (you can download it from our download site) to update the product.
Step 2: Import required GeneXus modules
The user must import 2 GeneXus modules (provided they are not already installed):
1 - SecurityAPICommons
2 - GeneXusJWT
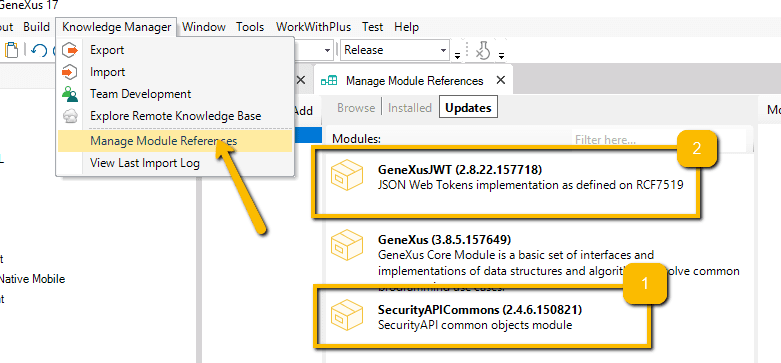
Step 3: Import new resources
The user must re-import the resources from the following 'xpz' file:
<GeneXus_Path>\UserControls\WorkWithPlusVideoCallWeb\WWPVideoCallWebResources.xml
Step 4: Add files to your deployment:
Sinch web client requires the registration of a Service Worker in order to work properly. Please note that Service Workers requires "https" protocol to be used (only localhost can be used with HTTP).
Among the resources imported on Step 3, a new "File" containing the Service Worker javascript is distributed.
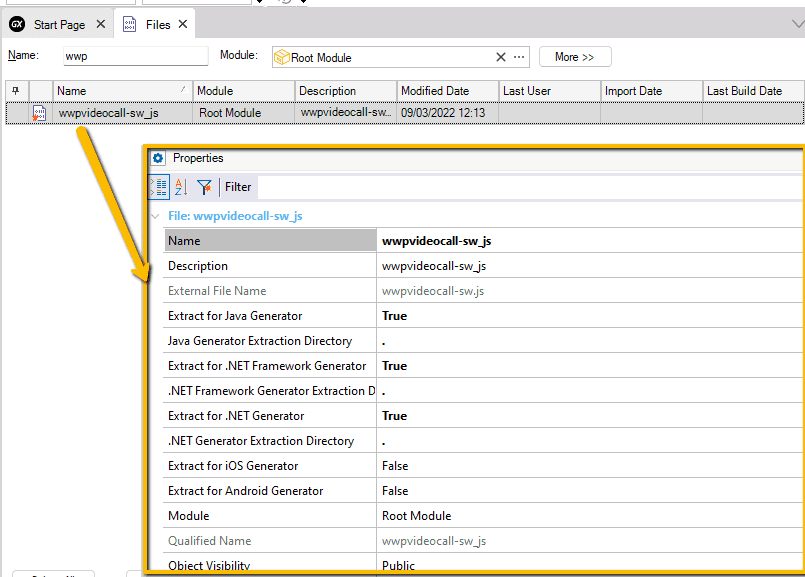
By default, this file will copy itself to the Web model automatically, but if you are using a Deployment Unit or "Deploy to Cloud" in Java you will need to add the file manually.
In case of using a deployment unit, simply add the file to all your deployment units.
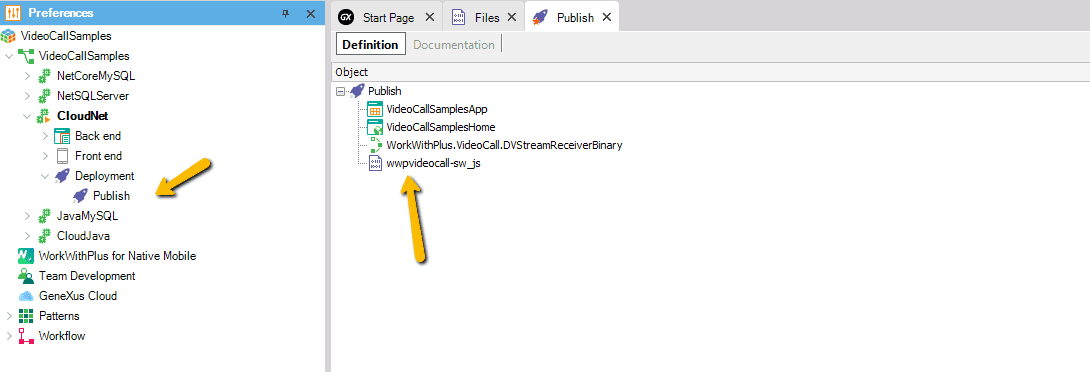
If you are using GeneXus "Deploy to Cloud" with the Java generator, you must manually copy the .js file to Tomcat's web app root. The file can be copied from the model's target path, e.g.: "<Model Path>\Web\wwpvideocall-sw.js"
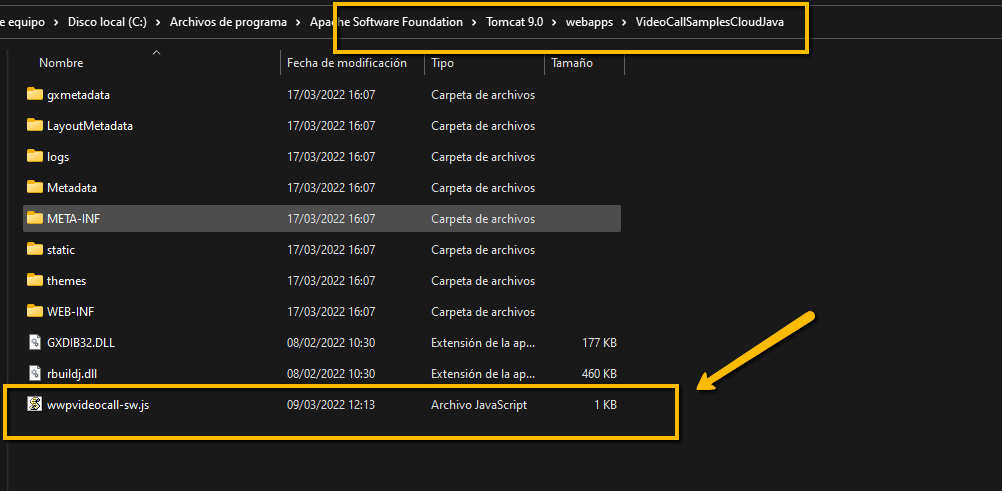
Step 5: Migrate your Panel/Web Panel code to match new requirements:
1.- Sinch SDK init.
In this version, Sinch requires a new 'Token' to be passed to the SDK in order to initialize.
The following changes were made to the User control, to adapt to the new features:
- The properties "API Key", "API Secret" and "API Host" were removed
- The method "initClient" was renamed and changed to "startVideoCallClient", and the parameters were changed, to accept the new initialization method.
Therefore, the initialization of the SDK must be migrated to the following:
Event Start
// Init your UI
// Get your SDK data. e.g: VideoCallSampleGetAPIData(&APIKey, &APISecret, &APIHost
// Get the new Token using the following procedure (LocalClientId should represent the current user):
&UserToken = VCGetAuthJWT(&LocalClientId, &APIKey, &APISecret, &ErrorMessage)
If &ErrorMessage.IsEmpty()
// start video client
VideoCallView.startVideoCallClient(&APIKey.ToString(), &APIHost.ToString(), &LocalClientId.ToString(), &UserToken.ToString())
VCRegisterActiveUser(&LocalClientId, ClientInformation.DeviceType)
Log.Debug(!"Video call is initializing")
Else
Log.Error(&ErrorMessage)
EndIf
EndEvent
1.- Sinch SDK init.
In this version, Sinch requires a new 'Token' to be passed in order to initialize.
The following changes were made to the User control, to adapt to the new features:
- The properties "API Key" and "Local User" were removed.
- The control doesn't initialize automatically on page load.
- A new method "startVideoCallClient" was added to replace the automatic initialization, and must be invoked by the user in the start event.
Therefore, the initialization of the SDK must be migrated to the following:
Event Start
// Init your UI
// Get your SDK data. e.g: VideoCallSampleGetAPIData(&APIKey, &APISecret, &APIHost
// Get the new Token using the following procedure (LocalClientId should represent the current user):
&UserToken = VCGetAuthJWT(&LocalClientId, &APIKey, &APISecret, &ErrorMessage)
If &ErrorMessage.IsEmpty()
VideoCallView.startVideoCallClient(&APIKey, &APIHost, &LocalClientId, &UserToken)
VCRegisterActiveUser(&LocalClientId, 9)
Log.Debug(!"Video call is initializing")
Else
Log.Error(&ErrorMessage)
EndIf
EndEvent
Due to new platform restrictions, video calls from mobile to web must be created using "Push Notifications".
But this mechanism is currently not supported by WorkWithPlus for VideoCall. This means it is not possible to initiate a video call from a Native Mobile application using the "UserControl.callUser()" method.
While we work on supporting such features, Video calls must be always initiated by the Web application.
If your application requires the Native Mobile user to start the video call, there are several ways to work around this restriction.
By default, WorkWithPlus for VideoCall provides some APIs that can be used for that matter:
Important: Please consider, that this solution uses GeneXus Server Cache API and Web Sockets.
Mobile Application
1 - After initializing the SDK, call the "VCRegisterActiveUser" procedure to register the user in the server and associate the platform (Android, iOS)
VideoCallView.startVideoCallClient(&APIKey.ToString(), &APIHost.ToString(), &LocalClientId.ToString(), &UserToken.ToString())
VCRegisterActiveUser(&LocalClientId, ClientInformation.DeviceType)
2 - Before Calling the remote user, call the "VCRegisterActionCall " procedure to check if it can be called directly. In case the remote client is a Web client, the procedure will fire a web push notification to signal the reverse call.
&CallAction = VCRegisterActionCall(&LocalClientId, &RemoteClientId)
If &CallAction = !"call"
VideoCallView.callTo(&RemoteClientId)
EndIf
3 - On the "CallReceived" event, you must check whether the call is a "common" call, or it is a reverse call, provoked by step 2
Event VideoCallView.CallReceived
If &CallAction = !"wait"
VideoCallView.answer() // Automatically answer reverse call
&CallAction = !""
Else
// Your current CallReceived Logic
EndIf
EndEvent
Web application
1 - After initializing the SDK, call the "VCRegisterActiveUser" procedure to register the user in the server and associate the platform (in this case, Web)
VideoCallView.startVideoCallClient(&APIKey.ToString(), &APIHost.ToString(), &LocalClientId.ToString(), &UserToken.ToString())
VCRegisterActiveUser(&LocalClientId, 9)
&HiddenClientId.SetEmpty()
2 - Web Panel must listen to web push notifications, and use them to initiate the call.
If you use Web Push notifications for other purposes, you should filter the information. You can check and modify the procedure "VCNotifyCallRequest" e.g. to add a custom prefix to the NotificationId.
In this example, we are using the variable "&HiddenClientId" which you must add to your WebForm, inside an Invisible Table, or at least set it as "&HiddenClientId.Visible=False"
Event OnMessage(&NotificationInfo)
&HiddenClientId = &NotificationInfo.Id
If &HiddenClientId.StartsWith(!"call:")
&HiddenClientId = &HiddenClientId.Substring(6)
Do 'OnCallReceived' // Your can use the "CallReceived" logic you already have or you could fire the videocall at the moment: VideoCallView.callUser(&HiddenClientId)
Else
&HiddenClientId.SetEmpty()
EndIf
EndEvent
3 - When the web user "Answers" the call, you must distinguish between a common call, and a reverse call
Event 'Answer'
If Not &HiddenClientId.IsEmpty()
VideoCallView.callUser(&HiddenClientId)
Else
VideoCallView.answer()
EndIf
Endevent
When using GeneXus deployment units in Java an error related to GeneXus JWT module will be displayed:
[com.genexus.servlet.ServletException: com.genexus.servlet.ServletException: java.lang.NoClassDefFoundError: com/genexus/commons/JWTOptions
at com.securityapi.genexusjwt.SdtJWTOptions.addheaderparameter(SdtJWTOptions.java:183)
...
java.lang.ClassNotFoundException: com.genexus.commons.JWTOptions
...
If this error is shown, it means GeneXus did not copy all the JWT module's necessary libraries in the deployed application.
You can check further details in the following GeneXus SAC: https://www.genexus.com/es/developers/websac?data=49833
To solve this problem, you will have to manually add the missing libraries to your deployment units. The missing files "version-number" usually depend on the GeneXus version you are using: https://wiki.genexus.com/commwiki/servlet/wiki?43980,GeneXus+JWT+Module
The following libraries correspond to GeneXus 17 Upgrade 8:
- bcpkix-jdk15on-1.69.jar
- java-jwt-3.10.3.jar
- GeneXusJWT-17.8.0.jar (17.8.0 stands for GeneXus 17 Upgrade 8)
- SecurityAPICommons-17.8.0.jar (17.8.0 stands for GeneXus 17 Upgrade 8)
To add these files to your deployment unit:
1 - Find the original .jar files in any of the following locations:
- <Target Environment>\Web\drivers
- <Local Tomcat Installation>\webapps\<Local WebApp>\WEB-INF\lib
2 - Add each file to the KB
3 - Set each file the property "Java Generator Extraction Directory"=".\WEB-INF\lib"
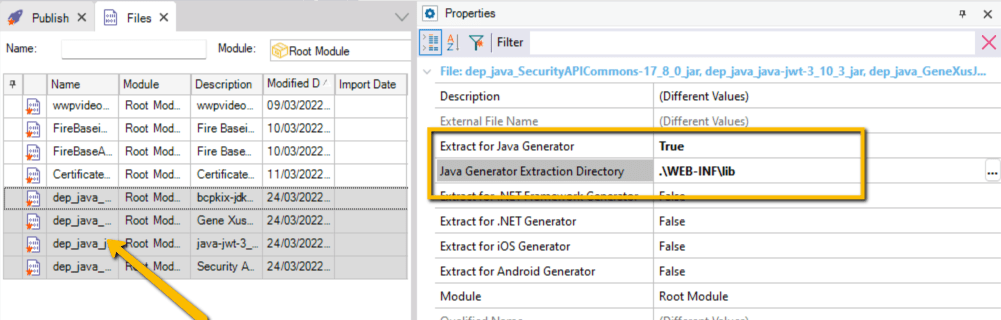
4 - Add files to the deployment unit
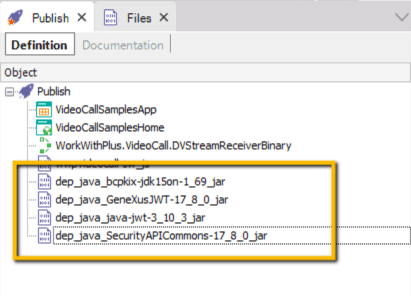
5 - Now you should be able to Build and Deploy your application
|